Fixing the "package command-line-arguments is not a main package" Error in Golang
Introduction
While learning Go, I encountered an interesting error:
package command-line-arguments is not a main package
At first, it was a bit confusing. I had written a simple Go program, but when I tried to run it, this error popped up.
After some digging, I figured out what was happening, why this error occurs, and how to fix it.
I’m sharing my learning experience here so that if you ever run into this issue, you’ll know exactly how to solve it.
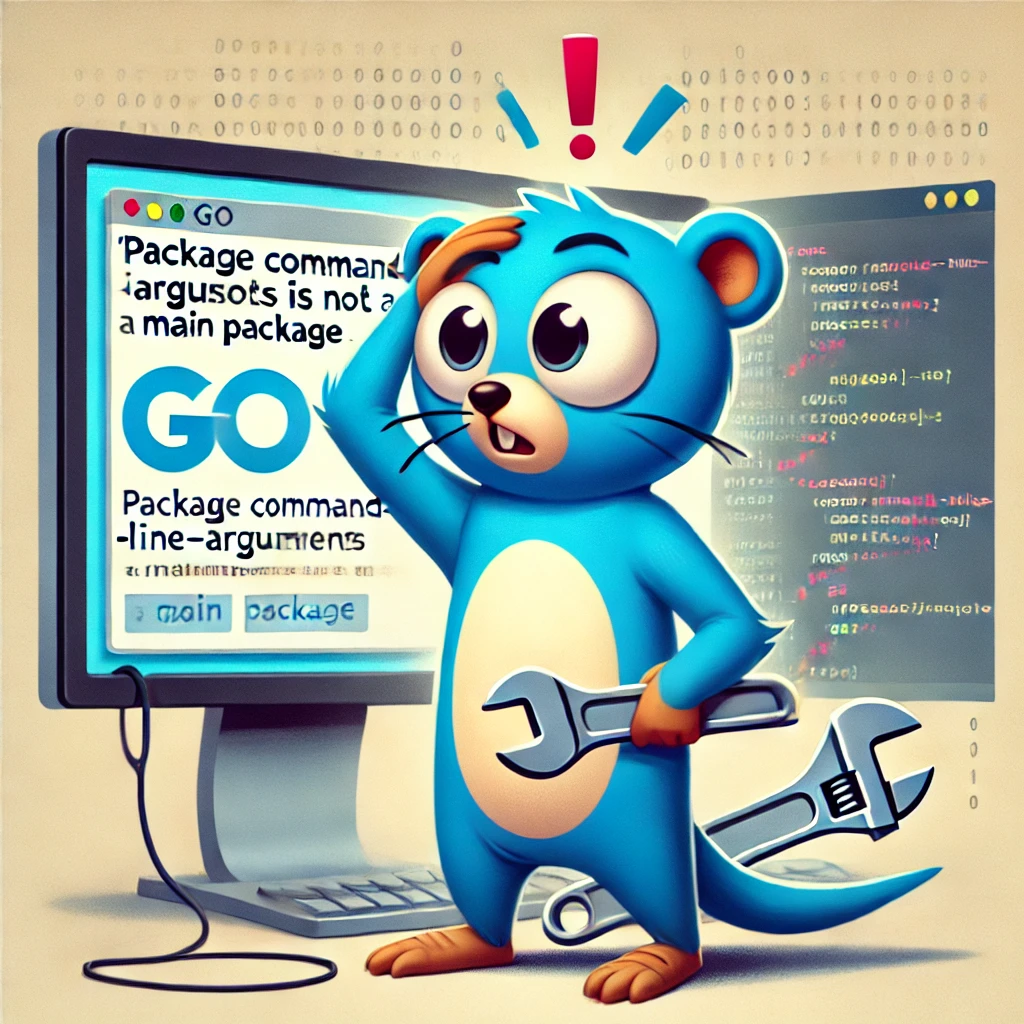
How I Got This Error
I was experimenting with Go and wrote a basic program like this:
package mypackage
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
Then, I tried to run it using:
go run example.go
And boom! I got this error:
package command-line-arguments is not a main package
At first, I wasn’t sure what this meant.
But after some research, I learned that this happens when Go doesn’t recognize the file as part of an executable package.
Why This Error Happens
Here’s what I figured out:
- The Go compiler expects an executable program to belong to the
main
package. - Since I named my package
mypackage
, Go didn’t treat it as a runnable program. - When using
go run
, Go compiles the file into a temporary binary and assigns it the namecommand-line-arguments
.
But if it’s not a main
package, it fails to generate an executable, leading to this error.
How I Fixed It
✅ Solution 1: Changing the Package to main
To fix the issue, I changed my code like this:
package main
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
Now, when I ran:
go run example.go
It worked perfectly! 🎉
✅ Solution 2: Using go build
for Other Packages
Later, I realized that if I wanted to structure my project with multiple packages, I shouldn’t use go run
directly on non-main packages.
For example, if I have a project like this:
myproject/
│── main.go
└── mypackage/
├── mypackage.go
Where mypackage.go
contains:
package mypackage
import "fmt"
func PrintMessage() {
fmt.Println("Hello from mypackage!")
}
And main.go
contains:
package main
import (
"mypackage"
)
func main() {
mypackage.PrintMessage()
}
I learned that instead of using go run
, I should build and run it like this:
go build -o myapp main.go
./myapp
This approach ensures that Go recognizes my packages properly and doesn’t assign command-line-arguments
as the package name.
Final Thoughts
This was a great learning experience for me.
I realized that Go has strict rules about package names, and understanding them helped me avoid this error in the future.
The key takeaways are:
✅ Always use package main
when writing an executable Go program.
✅ Use go build
instead of go run
when working with multiple packages.
✅ command-line-arguments
is just a temporary name Go gives to files that don’t belong to a proper package.
I hope my learning helps you avoid this confusion when working with Go. Happy coding! 🚀