How to Update All Go Modules with Examples: A Complete Guide for Developers
As I continue my journey with Go, one of the key challenges I encountered was managing dependencies efficiently.
Keeping Go modules up to date ensures stability, security, and compatibility with newer versions of packages.
Here’s what I learned along the way about updating all Go modules.
Understanding Go Modules
Go modules are the way Go handles dependencies.
They help define the project’s required packages, ensuring a consistent development environment.
The key files involved are:
- go.mod – Defines the module and dependencies.
- go.sum – Stores checksums of dependencies for verification.
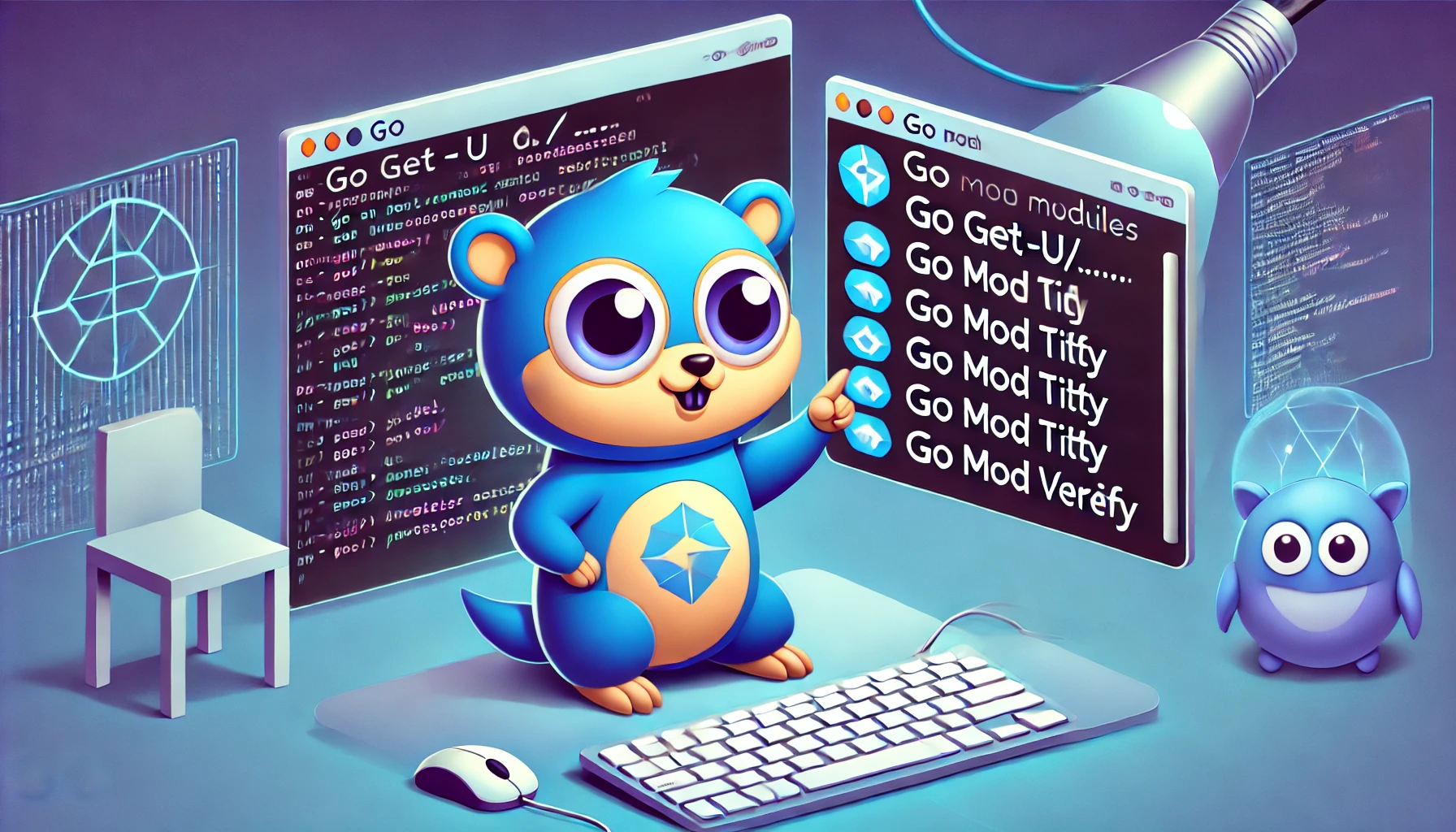
The Basic Update: go get -u ./...
When I first started updating dependencies, I used the following command:
go get -u ./...
This command updates all dependencies to their latest minor and patch versions.
It was a quick way to bring everything up to date without worrying about breaking changes introduced by major version upgrades.
Cleaning Up with go mod tidy
After updating dependencies, I found that my go.mod
and go.sum
files sometimes contained unused or redundant entries. Running:
go mod tidy
helped clean up unnecessary dependencies and ensured my project had only what was required.
Verifying Dependencies with go mod verify
To make sure everything was correctly downloaded and validated, I ran:
go mod verify
This confirmed that all dependencies matched their expected checksums, which reassured me that I wasn’t accidentally pulling in corrupted or modified packages.
Upgrading to Latest Major Versions
One thing I learned the hard way is that go get -u
only updates to the latest minor or patch versions, but it doesn’t automatically upgrade to newer major versions.
To upgrade to the latest major version, I needed to explicitly specify it, like this:
go get example.com/somepackage@latest
If I wanted to update all dependencies, including major versions, I used this handy command:
go list -m -u all | awk '{print $1}' | xargs -n 1 go get -u
This iterated over all dependencies and upgraded them to their latest versions.
Different Ways to Update Go Modules
As I explored Go further, I learned that go get -u
can be used in different ways depending on the use case:
go get -t -u ./...
: This walks all packages in the project and also downloads test files of these dependencies. In most cases, I found that I didn’t need this unless I was dealing with test dependencies explicitly.go get -u
: This updates dependencies only in the current directory. I found this useful for small single-package projects but preferredgo get -u ./...
for larger projects.go get -u specific.com/package
: This allows updating a single package or multiple specific packages (separated by spaces). I used this when I only wanted to update a particular dependency without affecting others.go get -u specific.com/package@version
: This works similarly but allows updating to a specific version. I found this helpful when a new major version introduced breaking changes, and I wanted to test a specific release.go get -u all
: This updates modules from the build list defined ingo.mod
. While it’s useful for listing outdated dependencies (go list -m -u all
), I found it not particularly useful for actual updates.
Testing After Updates
After updating dependencies, I always make sure my code still works as expected by running:
go build
and
go test ./...
This ensures that any breaking changes are caught early before deploying the application.
Hands-On Example: Updating Go Modules in a Sample Project
To put everything into practice, I created a simple Go project to experiment with updating modules. Here’s how I set it up:
Step 1: Initialize a Go Module
I started by creating a new project directory and initializing a Go module:
mkdir modules
cd modules
go mod init github.com/arungudelli/go-update-module-demo/modules
Step 2: Add Some Dependencies
Next, I installed a few popular Go packages:
go get github.com/gin-gonic/gin@v1.8.2
go get github.com/spf13/cobra@v1.6.1
go get golang.org/x/text@v0.5.0
These packages provided:
gin
– A web framework.cobra
– A CLI framework.golang.org/x/text
– Text processing utilities.
Step 3: Create a Simple Go File
Then, I created a main.go
file using these dependencies:
package main
import (
"fmt"
"github.com/gin-gonic/gin"
"github.com/spf13/cobra"
"golang.org/x/text/language"
)
func main() {
// Initialize Gin router
r := gin.Default()
r.GET("/", func(c *gin.Context) {
c.JSON(200, gin.H{"message": "Hello, Go Modules!"})
})
// Initialize Cobra CLI command
var rootCmd = &cobra.Command{
Use: "hello",
Short: "Prints a greeting message",
Run: func(cmd *cobra.Command, args []string) {
fmt.Println("Hello from Cobra CLI!")
},
}
// Use golang.org/x/text package
lang := language.English
fmt.Println("Default Language:", lang)
// Start Gin server in a goroutine
go r.Run(":8080")
// Execute Cobra CLI
rootCmd.Execute()
}
Step 4: Check for Available Updates
To see outdated dependencies, I ran:
go list -m -u all
It listed all the modules which are outdated.
github.com/cpuguy83/go-md2man/v2 v2.0.2 [v2.0.6]
github.com/creack/pty v1.1.9 [v1.1.24]
github.com/davecgh/go-spew v1.1.1
github.com/gin-contrib/sse v0.1.0 [v1.0.0]
github.com/gin-gonic/gin v1.8.2 [v1.10.0]
github.com/go-playground/assert/v2 v2.0.1 [v2.2.0]
github.com/go-playground/locales v0.14.0 [v0.14.1]
github.com/go-playground/universal-translator v0.18.0 [v0.18.1]
github.com/go-playground/validator/v10 v10.11.1 [v10.25.0]
github.com/goccy/go-json v0.9.11 [v0.10.5]
github.com/golang/protobuf v1.5.0 [v1.5.4] (deprecated)
github.com/google/go-cmp v0.5.5 [v0.7.0]
github.com/google/gofuzz v1.0.0 [v1.2.0]
github.com/inconshreveable/mousetrap v1.0.1 [v1.1.0]
github.com/json-iterator/go v1.1.12
github.com/kr/pretty v0.3.0 [v0.3.1]
github.com/kr/pty v1.1.1 [v1.1.8]
github.com/kr/text v0.2.0
github.com/leodido/go-urn v1.2.1 [v1.4.0]
github.com/mattn/go-isatty v0.0.16 [v0.0.20]
github.com/modern-go/concurrent v0.0.0-20180228061459-e0a39a4cb421 [v0.0.0-20180306012644-bacd9c7ef1dd]
github.com/modern-go/reflect2 v1.0.2
github.com/pelletier/go-toml/v2 v2.0.6 [v2.2.3]
github.com/pkg/diff v0.0.0-20210226163009-20ebb0f2a09e [v0.0.0-20241224192749-4e6772a4315c]
github.com/pmezard/go-difflib v1.0.0
github.com/rogpeppe/go-internal v1.8.0 [v1.14.1]
github.com/russross/blackfriday/v2 v2.1.0
github.com/spf13/cobra v1.6.1 [v1.9.1]
github.com/spf13/pflag v1.0.5 [v1.0.6]
github.com/stretchr/objx v0.5.0 [v0.5.2]
github.com/stretchr/testify v1.8.1 [v1.10.0]
github.com/ugorji/go v1.2.7 [v1.2.12]
github.com/ugorji/go/codec v1.2.7 [v1.2.12]
golang.org/x/crypto v0.0.0-20211215153901-e495a2d5b3d3 [v0.36.0]
golang.org/x/mod v0.6.0-dev.0.20220419223038-86c51ed26bb4 [v0.24.0]
golang.org/x/net v0.4.0 [v0.37.0]
golang.org/x/sys v0.3.0 [v0.31.0]
golang.org/x/term v0.3.0 [v0.30.0]
golang.org/x/text v0.5.0 [v0.23.0]
golang.org/x/tools v0.1.12 [v0.31.0]
golang.org/x/xerrors v0.0.0-20191204190536-9bdfabe68543 [v0.0.0-20240903120638-7835f813f4da]
google.golang.org/protobuf v1.28.1 [v1.36.5]
gopkg.in/check.v1 v1.0.0-20201130134442-10cb98267c6c
gopkg.in/errgo.v2 v2.1.0
gopkg.in/yaml.v2 v2.4.0
gopkg.in/yaml.v3 v3.0.1
Step 5: Update Dependencies
To update everything to the latest minor/patch versions:
go get -u ./...
And the above command gets the latest go modules.
go: downloading github.com/spf13/cobra v1.9.1
go: downloading golang.org/x/text v0.23.0
go: downloading github.com/gin-gonic/gin v1.10.0
go: downloading github.com/spf13/pflag v1.0.6
go: downloading golang.org/x/net v0.37.0
go: downloading github.com/mattn/go-isatty v0.0.20
go: downloading github.com/inconshreveable/mousetrap v1.1.0
go: downloading github.com/gin-contrib/sse v1.0.0
go: downloading github.com/goccy/go-json v0.10.5
go: downloading github.com/ugorji/go v1.2.12
go: downloading github.com/go-playground/validator/v10 v10.25.0
go: downloading google.golang.org/protobuf v1.36.5
go: downloading github.com/ugorji/go/codec v1.2.12
go: downloading github.com/pelletier/go-toml/v2 v2.2.3
go: downloading golang.org/x/sys v0.31.0
go: downloading github.com/modern-go/concurrent v0.0.0-20180306012644-bacd9c7ef1dd
go: downloading github.com/go-playground/validator v9.31.0+incompatible
go: downloading github.com/pelletier/go-toml v1.9.5
go: downloading golang.org/x/crypto v0.36.0
go: downloading github.com/go-playground/universal-translator v0.18.1
go: downloading github.com/leodido/go-urn v1.4.0
go: downloading github.com/go-playground/locales v0.14.1
go: downloading github.com/bytedance/sonic v1.11.6
go: downloading gopkg.in/yaml.v3 v3.0.1
go: downloading github.com/gabriel-vasile/mimetype v1.4.8
go: downloading github.com/bytedance/sonic v1.13.1
go: downloading github.com/cloudwego/base64x v0.1.4
go: downloading golang.org/x/arch v0.8.0
go: downloading github.com/twitchyliquid64/golang-asm v0.15.1
go: downloading github.com/bytedance/sonic/loader v0.1.1
go: downloading github.com/klauspost/cpuid/v2 v2.2.7
go: downloading golang.org/x/arch v0.15.0
go: downloading github.com/cloudwego/base64x v0.1.5
go: downloading github.com/bytedance/sonic/loader v0.2.4
go: downloading github.com/klauspost/cpuid/v2 v2.2.10
go: downloading github.com/klauspost/cpuid v1.3.1
go: downloading github.com/cloudwego/iasm v0.2.0
go: added github.com/bytedance/sonic v1.13.1
go: added github.com/bytedance/sonic/loader v0.2.4
go: added github.com/cloudwego/base64x v0.1.5
go: added github.com/cloudwego/iasm v0.2.0
go: added github.com/gabriel-vasile/mimetype v1.4.8
go: upgraded github.com/gin-contrib/sse v0.1.0 => v1.0.0
go: upgraded github.com/gin-gonic/gin v1.8.2 => v1.10.0
go: upgraded github.com/go-playground/locales v0.14.0 => v0.14.1
go: upgraded github.com/go-playground/universal-translator v0.18.0 => v0.18.1
go: upgraded github.com/go-playground/validator/v10 v10.11.1 => v10.25.0
go: upgraded github.com/goccy/go-json v0.9.11 => v0.10.5
go: upgraded github.com/inconshreveable/mousetrap v1.0.1 => v1.1.0
go: added github.com/klauspost/cpuid/v2 v2.2.10
go: upgraded github.com/leodido/go-urn v1.2.1 => v1.4.0
go: upgraded github.com/mattn/go-isatty v0.0.16 => v0.0.20
go: upgraded github.com/modern-go/concurrent v0.0.0-20180228061459-e0a39a4cb421 => v0.0.0-20180306012644-bacd9c7ef1dd
go: upgraded github.com/pelletier/go-toml/v2 v2.0.6 => v2.2.3
go: upgraded github.com/spf13/cobra v1.6.1 => v1.9.1
go: upgraded github.com/spf13/pflag v1.0.5 => v1.0.6
go: added github.com/twitchyliquid64/golang-asm v0.15.1
go: upgraded github.com/ugorji/go/codec v1.2.7 => v1.2.12
go: added golang.org/x/arch v0.15.0
go: upgraded golang.org/x/crypto v0.0.0-20211215153901-e495a2d5b3d3 => v0.36.0
go: upgraded golang.org/x/net v0.4.0 => v0.37.0
go: upgraded golang.org/x/sys v0.3.0 => v0.31.0
go: upgraded golang.org/x/text v0.5.0 => v0.23.0
go: upgraded google.golang.org/protobuf v1.28.1 => v1.36.5
PS D:\Blogging\go-learning\go-update-module-demo\modules> go mod tidy
go: downloading github.com/stretchr/testify v1.10.0
go: downloading github.com/google/go-cmp v0.5.5
go: downloading github.com/go-playground/assert/v2 v2.2.0
go: downloading gopkg.in/check.v1 v0.0.0-20161208181325-20d25e280405
go: downloading github.com/davecgh/go-spew v1.1.1
go: downloading golang.org/x/xerrors v0.0.0-20191204190536-9bdfabe68543
go: downloading github.com/pmezard/go-difflib v1.0.0
To clean up unused dependencies:
go mod tidy
For verifying module integrity:
go mod verify
output:
all modules verified
Step 6: Update Specific Modules
For major version updates, I used:
go get github.com/gin-gonic/gin@latest
Step 7: Testing the Updates
After updating, I built and tested the project:
go build
./go-update-demo
Everything worked as expected, proving that managing and updating Go modules can be streamlined with these commands.
Here is the Github link for the example
https://github.com/arungudelli/go-update-module-demo
Key Takeaways
- Use
go get -u ./...
to update all dependencies to the latest compatible versions. - Run
go mod tidy
to clean up unused dependencies. - Verify dependencies with
go mod verify
to ensure integrity. - Manually update major versions using
go get package@latest
when necessary. - Different
go get -u
options exist for updating specific packages, test dependencies, or entire module lists. - Always test your code after updates with
go build
andgo test ./...
.
Learning to manage Go modules effectively has helped me avoid dependency issues and ensure my projects remain up-to-date. Hopefully, my experience can help others streamline their Go module updates as well!